Python Programming
- Description
- Curriculum
- Reviews
- Grade
Python programming finds applications across a wide range of domains and industries due to its versatility, simplicity, and extensive ecosystem of libraries and frameworks. Here are some common applications of Python programming:
-
Web Development:
- Python web frameworks like Django, Flask, and Pyramid are widely used for building web applications and APIs.
- Python’s simplicity and readability make it easy to develop and maintain web applications.
-
Data Science and Data Analysis:
- Python, along with libraries like NumPy, Pandas, Matplotlib, and SciPy, is extensively used for data manipulation, exploration, visualization, and analysis.
- Python’s simplicity and rich ecosystem make it the preferred language for data science and machine learning projects.
-
Artificial Intelligence and Machine Learning:
- Python, along with libraries like TensorFlow, Keras, PyTorch, and scikit-learn, is widely used for building and training machine learning models.
- Python’s simplicity, readability, and extensive libraries make it suitable for research, prototyping, and production deployment of AI and ML models.
-
Scientific Computing:
- Python is used for scientific computing and numerical simulations in fields like physics, chemistry, biology, and engineering.
- Libraries like NumPy, SciPy, SymPy, and matplotlib provide tools for numerical computations, symbolic mathematics, and visualization.
-
Automation and Scripting:
- Python’s simplicity and cross-platform compatibility make it ideal for automating repetitive tasks, system administration, and scripting.
- Python scripts can automate tasks such as file manipulation, data processing, web scraping, and batch processing.
-
Game Development:
- Python, along with libraries like Pygame and Panda3D, is used for developing 2D and 3D games.
- Python’s ease of use and rapid development capabilities make it suitable for game prototyping and development.
-
Desktop GUI Applications:
- Python, with libraries like Tkinter, PyQt, and wxPython, is used for building desktop GUI applications.
- Python’s simplicity and cross-platform compatibility make it suitable for developing desktop applications with graphical user interfaces.
-
Web Scraping and Crawling:
- Python’s libraries like BeautifulSoup and Scrapy are used for web scraping and crawling to extract data from websites.
- Python’s simplicity and powerful libraries make it ideal for scraping and analyzing web data.
-
Network Programming:
- Python’s
socket
library and frameworks like Twisted and asyncio are used for network programming, including building servers, clients, and network protocols. - Python’s simplicity and extensive networking libraries make it suitable for developing networking applications.
- Python’s
These are just a few examples of the diverse applications of Python programming. Its simplicity, versatility, and rich ecosystem of libraries make it a popular choice for developers across various domains and industries.
-
1Introduction to Python Programming LanguagePreview 7
Python is a high-level, interpreted programming language known for its simplicity and readability. It supports multiple programming paradigms, including procedural, object-oriented, and functional programming. Python's extensive standard library and vibrant ecosystem of third-party packages make it suitable for a wide range of applications, such as web development, data analysis, machine learning, automation, and more. Its dynamic typing and straightforward syntax make it an excellent choice for both beginners and experienced developers.
-
2Installation of Software10
Here we will learn how to install Python IDE Software.
-
3Comment in Python Programming4
Here we will learn how to comment in Python Programming.
-
4Python Variables7
variables are used to store data that can be manipulated and retrieved later
-
5Python Operators7
Python provides a rich set of operators that allow you to perform various operations on variables and values.
-
6Collecting User Data in Python7
Here we will learn how to collect the user input data.
-
8If Statement5
The
if
statement in Python is used for conditional execution. -
9if-elif-else-statement13
We will learn how to code using if-elif-else statement in python.
-
10Grading system project12
We will be building a grading system with all knowledge we have so far...
-
11Login Module in Python Programming13
We will learn about the login Module in Python.
-
12For-Loop in python26
The
for
loop in Python is used to iterate over a sequence. -
13For Loop 215
The
for
loop in Python is used to iterate over a sequence. -
14For Loop 311
The
for
loop in Python is used to iterate over a sequence. -
15For Loop 426
The
for
loop in Python is used to iterate over a sequence. -
16While Loop14
The
while
loop in Python is used to repeatedly execute a block of code as long as a given condition is true. -
17Break statement10
The
break
statement in Python is used to terminate the execution of a loop prematurely -
18Continue Statement6
The
continue
statement in Python is used to skip the rest of the code inside a loop for the current iteration and proceed to the next iteration. -
19Fibonnaci series11
The Fibonacci series is a sequence of numbers where each number is the sum of the two preceding ones, usually starting with 0 and 1.
-
20Python QuizText lesson
Quiz
-
21Python Strings 19
Strings in Python are sequences of characters enclosed within single quotes (
' '
), double quotes (" "
), or triple quotes (''' '''
or""" """
). -
22Python String 28
Strings in Python are sequences of characters enclosed within single quotes (
' '
), double quotes (" "
), or triple quotes (''' '''
or""" """
). -
23Python String 311
Strings in Python are sequences of characters enclosed within single quotes (
' '
), double quotes (" "
), or triple quotes (''' '''
or""" """
). -
24Boolean String Method5
Boolean is a data type that has two possible values:
True
andFalse
. -
25Python String Split10
Learn how to use the String Split.
-
26Python String Join6
The
join()
method in Python is used to concatenate the elements of an iterable (like a list or tuple) into a single string. -
27String Replace in Python Programming4
The
replace()
method in Python is used to create a new string by replacing occurrences of a specified substring with another substring.
-
28Errors in Python Programming5
Errors in Python programming can occur for various reasons, including syntax errors, runtime errors, and logical errors.
-
29Try-Except in Python Programming9
-
30Try-except-else-finally10
The
try-except
block is used to handle exceptions gracefully. -
31Multiple Errors in Python4
You can handle multiple exceptions by adding multiple
except
blocks or by specifying multiple exception types in a singleexcept
block.
-
32List in Python ProgrammingVideo lesson
-
33List Slice in Python Programming6
List slicing allows you to extract a sublist (slice) from a list using slicing notation.
-
34List Method in Python Programming7
Lists are versatile data structures with built-in methods for manipulation.
-
35Tuple in Python11
-
36Tuple Slicing in Python10
Tuple slicing in Python works similarly to list slicing.
-
37Tuple unpacking in python10
Tuple unpacking, also known as iterable unpacking, is a powerful feature in Python that allows you to assign the elements of a tuple.
-
38Dictionaries in python16
Dictionary is a mutable, unordered collection of key-value pairs
-
39Nested Dictionary in Python14
Nested dictionaries in Python are dictionaries that contain other dictionaries as their values.
-
40Dictionary conversion8
Here we learn how to convert dictionary to list or to a tuple.
-
41Python Quiz 2Text lesson
Python Level 2 Quiz
-
42Creating Modules in Python10
Creating a module in Python allows you to organize your code into reusable files.
-
43Inbuilt Python Modules10
Python comes with a rich standard library of inbuilt modules that provide a wide range of functionalities.
-
44Functions in Python11
Functions in Python are blocks of reusable code that perform a specific task.
-
45Kwargs in Python Function10
kwargs
stands for "keyword arguments." It's a special syntax in Python that allows you to pass a variable number of keyword arguments to a function. -
46Lambda Function in Python8
Lambda function is a small anonymous function defined using the
lambda
keyword. -
47Pass in Python3
pass
is a keyword that is used as a placeholder where syntactically a statement is required, but no action needs to be taken. -
48Recursive Function13
A recursive function in Python is a function that calls itself within its own definition.
-
49Python Quiz 31 questions
-
50Calendar in Python13
calendar
module provides various functions to work with calendars. -
51Calendar Module Project13
Here we will embark on a Project using the Calendar Module.
-
52Time Module in Python8
The
time
module in Python provides various time-related functions. -
53Time Module 26
The
time
module in Python provides various time-related functions. -
54Time directive7
Directives allow you to create or parse date/time strings in various formats
-
55Datetime Date in Python8
datetime
module provides classes for manipulating dates and times in both simple and complex ways. -
56Progress Bar Project15
Here we will be developing a basic progress bar concept.
-
57List Comprehension Basics10
List comprehensions are a concise and powerful way to create lists in Python.
-
58Nested List Comprehension9
Nested list comprehensions allow you to create lists of lists (or other nested data structures) using a compact syntax.
-
59List Comprehension Examples10
Here we will explore more examples on List Comprehension.
-
60Map-Filter-Reduce in List Comprehension13
map()
,filter()
, andreduce()
are built-in functions for functional programming. While they're not directly implemented as list comprehensions. -
61Matrix Transpose in Python9
Here we will learn how to Transpose Matrix in Python.
-
62Text Extraction Exercise5
Here we will be performing an Excercise on Text Extraction techniques in Python.
-
63Python Quiz 3Text lesson
Python Level 3 Quiz
-
64Map Function9
the
map()
function is used to apply a specified function to each item in an iterable (like a list) and return a new iterable with the results. -
65Filter Function7
The
filter()
function is used to filter elements from an iterable (e.g., a list) based on a function's result. -
66Reduce Function9
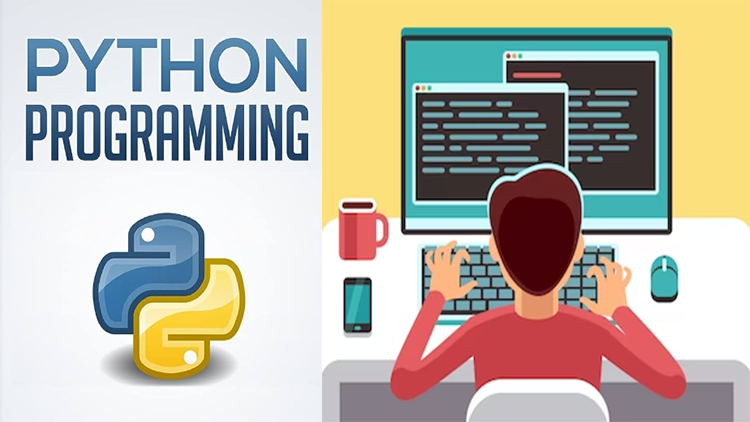
Basic Microsoft packages like Word, Excel.
Archive
Working hours
Monday | 9:30 am - 6.00 pm |
Tuesday | 9:30 am - 6.00 pm |
Wednesday | 9:30 am - 6.00 pm |
Thursday | 9:30 am - 6.00 pm |
Friday | 9:30 am - 5.00 pm |
Saturday | Closed |
Sunday | Closed |